At work a funny thing happens. I love working with computers, I think I’m a lucky guy. At work though I have so many distractions that I can’t get work done. For every distraction it drains my attention on what I’m doing, and when it exceeds a certain level then I’m not very useful.
When I go home and rest for a while, I’m drained, I don’t want to do much, it would be so easy to turn on the TV. Sometimes I go for a walk, but I’ll drag along, it’s been a long day and I just want to rest.
The funny thing that happens, is that I go sit at the computer and do something as simple as work on some basic Python challenges and I find it rejuvenating. I find following along a good course like Dr Angela Yu’s 100 projects in Python is a perfect blend of guided meditation and problem solving that it gets me out of that slump. Weird.
Here are my notes from the day:
Day 2 – Data Types
June 22, 2022 – Coding Rooms Exercises Day 2 (Lessons 1,2,3) Day 3 (1,2)
BMI-calculator.py
We played around with strings. Numbers as strings. Typecasting strings to integers or floats.
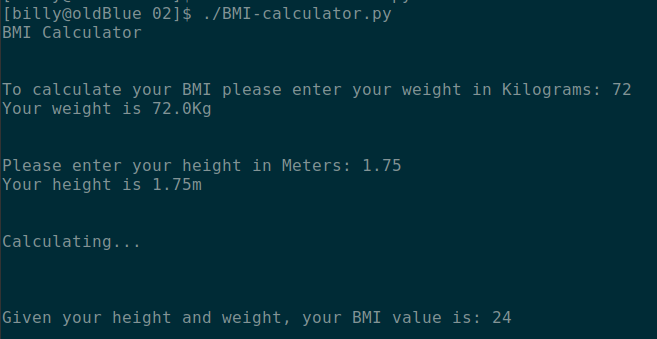
To declare a variable as a data type use:
varname = int(5)
But this will be overwritten when a new assignmentvarname = input("New Value:")
Will change the data type back to a string, even if a number is entered.
So I need to validate the data type after an input.varname = int(varname)
Formatting strings
I thought I would be able to use printf style formatting, there is a Python way using the .format() method and there is a printf method. Kinda weird either way.
age = input("What is your current age?")
age = int(age)
years_till_90 = 90 - age
age_in_months = years_till_90 * 12
age_in_weeks = years_till_90 * 52
age_in_days = years_till_90 * 365
These next lines produce the same result. The python way and the old printf way. The Python way is a bit more friendly for type conversion.
print('You have {} days, {} weeks, and {} months left.'.format(age_in_days, age_in_weeks, age_in_months))
print('You have %d days, %d weeks, and %d months left.' % (age_in_days, age_in_weeks, age_in_months))
print(f'You have {age_in_days} days, {age_in_weeks} weeks, and {age_in_months} months left.')
// this one is the most readable and likely the new standard. (Note the 'f' )
Operators
The standard operators apply: + – / * == != < <= >= >
Logic operators are words: and, or
maths
Standard operators built into python
rounding numbers round(23.2552, 2) will round two decimal places
modulo operator % 4 % 2 will result in 0. No remainder dividing 4 by 2. 5 % 2 will result 1
For more advanced commands like ceil, or floor, import the math module
Day 2 Assignment 3 – BMI Calculator 2
I struggled to pass the tests at first, due to my spelling of slightly vs slighty, and almost invisible difference in the code when you are looking for syntax differences.
Posted to twitter here: https://twitter.com/teknoBilly/status/1539827653091692544